Java SpringBoot MongoPlus 使用MyBatisPlus的方式,优雅的操作MongoDB
- 介绍
- 特性
- 安装
- 新建SpringBoot工程
- 引入依赖
- 配置文件
- 使用
- 新建实体类
- 创建Service
- 测试类进行测试
- 新增方法
- 查询方法
- 官方网站
- 获取本项目案例代码
介绍
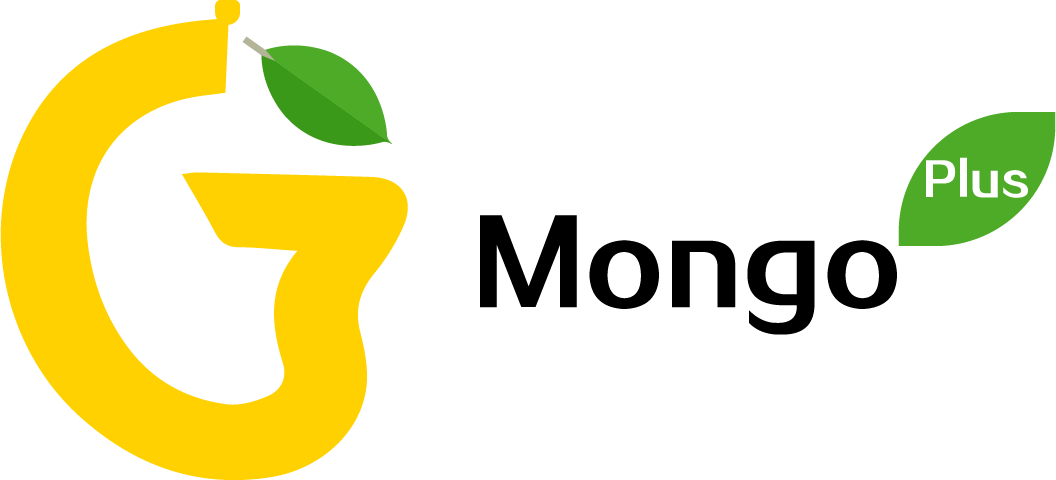
Mongo-Plus(简称 MP)是一个 MongoDB 的操作工具,可和现有mongoDB框架结合使用,为简化开发、提高效率而生。
特性
- 无侵入:只做增强不做改变,引入它不会对现有工程产生影响,如丝般顺滑
- 损耗小:启动即会自动注入基本 CURD,性能基本无损耗,直接面向对象操作
- 强大的 CRUD 操作:通用 Service,仅仅通过少量配置即可实现单表大部分 CRUD 操作,更有强大的条件构造器,满足各类使用需求
- 支持 Lambda 形式调用:通过 Lambda 表达式,方便的编写各类查询条件,无需再担心字段写错
- 支持主键自动生成:支持多达 5 种主键策略(内含分布式唯一 ID 生成器 - Sequence),可自由配置,完美解决主键问题
- 支持自定义全局通用操作:支持全局通用方法注入
- 支持无实体类情况下的操作
- 支持动态数据源
- 支持逻辑删除、防止全集合更新和删除、自动填充等等功能
安装
新建SpringBoot工程
采用的是IDEA新建的工程
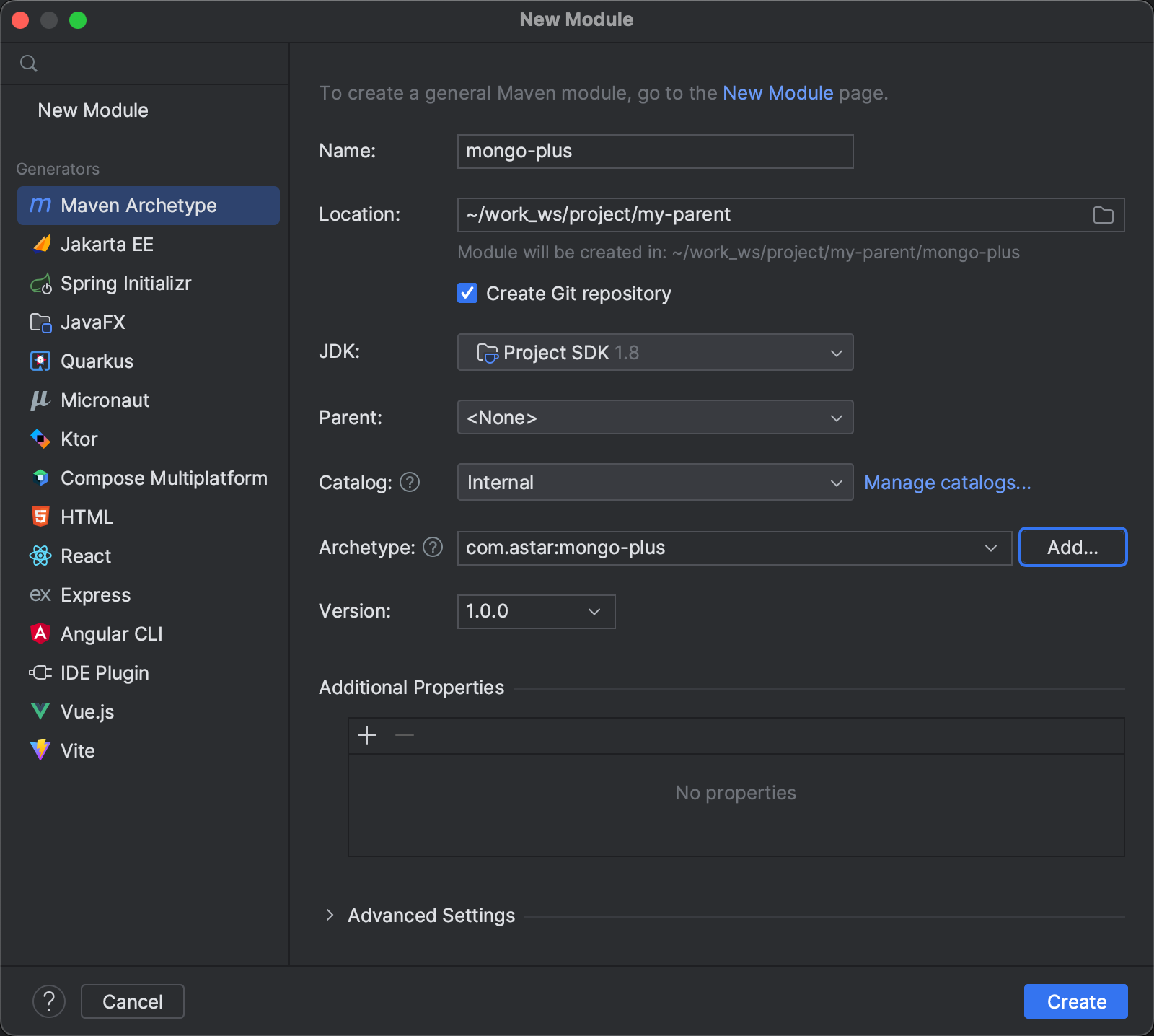
引入依赖
pom文件增加以下内容:
全部增加在project标签下面
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.13</version>
<relativePath/>
</parent>
<dependencies>
<!-- mongo-plus依赖 -->
<dependency>
<groupId>com.gitee.anwena</groupId>
<artifactId>mongo-plus-boot-starter</artifactId>
<version>2.0.9.3</version>
</dependency>
<!-- boot容器依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- 测试依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
</dependency>
<!-- lombok依赖 -->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
<!-- hutool工具依赖 -->
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.7.5</version>
</dependency>
</dependencies>
增加完后执行下载包命令
mvn clean install
最后重新加载maven依赖
配置文件
mongo-plus:
data:
mongodb:
host: 127.0.0.1 #ip
port: 27017 #端口
database: demo #数据库名
username: root #用户名,没有可不填(若账号中出现@,!等等符号,不需要再进行转码!!!)
password: root #密码,同上(若密码中出现@,!等等符号,不需要再进行转码!!!)
authenticationDatabase: admin #验证数据库
connectTimeoutMS: 50000 #在超时之前等待连接打开的最长时间(以毫秒为单位)
log: true
配置自己的数据库以及账号密码
使用
新建实体类
java">@Data
@CollectionName("astarDemo")
public class AstarDemoEntity {
/**
* 使用ID注解,标注此字段为MongoDB的_id,或者继承BaseModelID类
*/
@ID(type = IdTypeEnum.ASSIGN_ID)
private String id;
private String name;
private int age;
private String email;
}
创建Service
编写Service下的AstarDemoService和实现类AstarDemoServiceImpl,像MyBatisPlus一样
java">public interface AstarDemoService extends IService<AstarDemoEntity> {
}
java">public class AstarDemoServiceImpl extends ServiceImpl<AstarDemoEntity> implements AstarDemoService {
}
测试类进行测试
新增方法
java">@ContextConfiguration(classes = MongoPlusApp.class)
@SpringBootTest
public class AstarDemoTest {
@Autowired
private AstarDemoService astarDemoService;
@Test
public void insert() {
AstarDemoEntity entity = new AstarDemoEntity();
entity.setName("一颗星");
entity.setAge(111);
entity.setEmail("xxx@aaa.email");
astarDemoService.save(entity);
}
}
运行测试
控制台已经把日志给打印出来了
接下来我们看数据库是否存在
数据库中也存在数据
查询方法
java">@Test
public void query() {
LambdaQueryChainWrapper<AstarDemoEntity> lambdaQuery = astarDemoService.lambdaQuery();
// age为111的
lambdaQuery.eq(AstarDemoEntity::getAge, 111);
List<AstarDemoEntity> list = astarDemoService.list(lambdaQuery);
list.forEach(System.out::println);
}
官方网站
官方网址
获取本项目案例代码
获取本项目代码
公粽号:一颗星宇宙
发送mongo
获取